반응형
Retrofit을 이용한 REST API 활용 방법
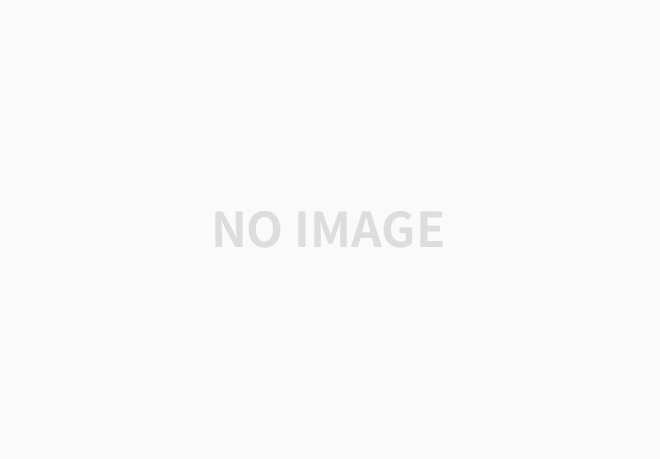
개요
Retrofit은 Android 및 Java 애플리케이션에서 REST API를 호출하기 위해 널리 사용되는 타입 안전 HTTP 클라이언트입니다. 이 글에서는 Retrofit을 이용하여 REST API를 호출하는 방법과 함께 발생할 수 있는 오류 상황과 해결 방법을 소개합니다.
1. 의존성 추가
프로젝트에 Retrofit과 OkHttp를 포함시키기 위해 build.gradle 파일에 다음과 같은 종속성을 추가합니다.
groovy
dependencies {
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
implementation 'com.squareup.okhttp3:okhttp:4.9.0'
}
2. API 인터페이스 정의
API 엔드포인트를 정의합니다. 예를 들어, 사용자 정보를 쿼리 파라미터로 전달하여 요청하는 경우를 보여드립니다.
java
import retrofit2.Call;
import retrofit2.http.GET;
import retrofit2.http.Query;
public interface ApiService {
@GET("users")
Call<UserResponse> getUser(@Query("id") int userId);
}
3. 데이터 모델 정의
API 응답을 매핑할 데이터 모델 클래스를 정의합니다.
java
public class UserResponse {
private int id;
private String name;
private String email;
// Getters and Setters
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
4. Retrofit 인스턴스 생성
OkHttp 클라이언트를 설정하고, Retrofit에 통합합니다.
java
import okhttp3.OkHttpClient;
import okhttp3.logging.HttpLoggingInterceptor;
import retrofit2.Retrofit;
import retrofit2.converter.gson.GsonConverterFactory;
public class RetrofitClient {
private static final String BASE_URL = "https://api.example.com/";
private static Retrofit retrofit;
public static Retrofit getRetrofitInstance() {
if (retrofit == null) {
// HttpLoggingInterceptor 설정 (옵션)
HttpLoggingInterceptor logging = new HttpLoggingInterceptor();
logging.setLevel(HttpLoggingInterceptor.Level.BODY);
// OkHttpClient 설정
OkHttpClient okHttpClient = new OkHttpClient.Builder()
.addInterceptor(logging) // 로깅 인터셉터 추가
.build();
// Retrofit 인스턴스 생성
retrofit = new Retrofit.Builder()
.baseUrl(BASE_URL)
.client(okHttpClient) // 커스텀 OkHttpClient 설정
.addConverterFactory(GsonConverterFactory.create())
.build();
}
return retrofit;
}
public static ApiService getApiService() {
return getRetrofitInstance().create(ApiService.class);
}
}
5. API 호출 및 응답 처리
API를 호출하고 응답을 처리하는 코드를 작성합니다.
java
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
public class Main {
public static void main(String[] args) {
ApiService apiService = RetrofitClient.getApiService();
Call<UserResponse> call = apiService.getUser(1);
call.enqueue(new Callback<UserResponse>() {
@Override
public void onResponse(Call<UserResponse> call, Response<UserResponse> response) {
if (response.isSuccessful()) {
UserResponse user = response.body();
System.out.println("ID: " + user.getId());
System.out.println("Name: " + user.getName());
System.out.println("Email: " + user.getEmail());
} else {
System.err.println("Request failed. Code: " + response.code());
}
}
@Override
public void onFailure(Call<UserResponse> call, Throwable t) {
t.printStackTrace();
}
});
}
}
오류 상황과 해결 방법
- NoClassDefFoundError: com/google/gson/Gson
gradle
implementation 'com.google.code.gson:gson:2.8.7'
- 이 오류는 Gson 라이브러리가 프로젝트에 포함되지 않았을 때 발생합니다. Gradle 파일에 다음과 같이 Gson 종속성을 추가하여 해결할 수 있습니다.
- NoClassDefFoundError: okio/Sink
gradle
implementation 'com.squareup.okio:okio:2.10.0'
- 이 오류는 Okio 라이브러리가 프로젝트에 포함되지 않았을 때 발생합니다. Gradle 파일에 다음과 같이 Okio 종속성을 추가하여 해결할 수 있습니다.
- Request failed. Code: 404
- 이 오류는 요청한 리소스를 찾을 수 없을 때 발생합니다. API 엔드포인트와 쿼리 파라미터가 올바른지 확인하십시오.
결론
Retrofit과 OkHttp를 사용하여 REST API를 호출하는 방법과 함께, 발생할 수 있는 일반적인 오류와 그 해결 방법을 소개했습니다. 이 글이 Retrofit을 활용하는 데 도움이 되길 바랍니다.
반응형
'갑을병정이야기' 카테고리의 다른 글
Remote Operations와 DxDataGrid를 활용한 웹 애플리케이션 예제 (1) | 2024.12.17 |
---|---|
Java와 함께 하는 koin(feat kotlin), json 활용 이야기 (1) | 2024.12.14 |
DevExpress dxDataGrid 사용하기: 사용자 입력과 날짜 형식 변환 (1) | 2024.10.27 |
Visual SVN에서 변경 이력 및 내역 확인하기 (1) | 2024.10.01 |
리눅스에서 /etc/group 파일에 사용자 추가하기 (1) | 2024.09.27 |