안녕하세요! 오늘은 Jetpack Compose와 com.afollestad.material-dialogs 라이브러리를 사용하여 비밀번호 입력 다이얼로그를 구현하는 방법에 대해 알아보겠습니다. Jetpack Compose는 최신 Android UI 툴킷으로, 선언형 프로그래밍 모델을 사용하여 UI를 효율적으로 구축할 수 있습니다. com.afollestad.material-dialogs는 다양한 유형의 다이얼로그를 쉽게 만들 수 있게 해주는 라이브러리입니다.
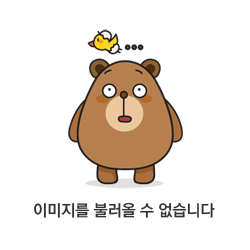
사전 준비
먼저, 프로젝트의 build.gradle 파일에 다음 의존성을 추가해야 합니다.
dependencies {
implementation "com.afollestad.material-dialogs:core:3.3.0"
implementation "com.afollestad.material-dialogs:input:3.3.0"
}
위의 의존성을 추가한 후, Gradle을 동기화(Sync)합니다.
비밀번호 입력 다이얼로그 구현
이제 Jetpack Compose에서 com.afollestad.material-dialogs 라이브러리를 사용하여 비밀번호 입력 다이얼로그를 구현하는 방법을 단계별로 살펴보겠습니다.
1. MainActivity 설정
먼저 MainActivity를 설정합니다. Jetpack Compose의 setContent를 사용하여 UI를 설정합니다.
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.*
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import com.example.myapp.ui.theme.MyAppTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyAppTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
PasswordDialogExample()
}
}
}
}
}
2. PasswordDialogExample 컴포저블
PasswordDialogExample 컴포저블은 버튼을 클릭할 때 비밀번호 입력 다이얼로그를 표시합니다. 다이얼로그가 닫히면 입력된 비밀번호를 표시합니다.
@Composable
fun PasswordDialogExample() {
var showDialog by remember { mutableStateOf(false) }
var password by remember { mutableStateOf("") }
if (showDialog) {
PasswordInputDialog(
onDismiss = { showDialog = false },
onPasswordEntered = { enteredPassword ->
password = enteredPassword
showDialog = false
}
)
}
Button(onClick = { showDialog = true }) {
Text("Show Password Dialog")
}
if (password.isNotEmpty()) {
Text("Entered Password: $password")
}
}
3. PasswordInputDialog 컴포저블
PasswordInputDialog 컴포저블은 Material Dialogs를 사용하여 비밀번호 입력 다이얼로그를 생성합니다. 비밀번호 입력이 완료되면 onPasswordEntered 콜백을 호출하고, 다이얼로그가 닫힙니다.
@Composable
fun PasswordInputDialog(
onDismiss: () -> Unit,
onPasswordEntered: (String) -> Unit
) {
val context = LocalContext.current
LaunchedEffect(Unit) {
MaterialDialog(context).show {
title(text = "Enter Password")
input(
hint = "Password",
inputType = InputType.TYPE_CLASS_TEXT or InputType.TYPE_TEXT_VARIATION_PASSWORD
) { dialog, text ->
onPasswordEntered(text.toString())
}
positiveButton(text = "Submit") {
it.dismiss()
}
negativeButton(text = "Cancel") {
it.dismiss()
}
lifecycleOwner(LocalLifecycleOwner.current)
}.setOnDismissListener {
onDismiss()
}
}
}
4. Xml Layout의 구성
input 을 사용하는 경우에는 라이브러리 제공자의 github 에서 참조 하여 xml layout 을 복사해 주어야 합니다. 이유는 사용하는 라이브러리가 xml layout 을 기반으로 하고 있어서 그런 부분이 있습니다. 자세한 내용은 원작자의 github 을 참고 하세요.
material-dialogs/documentation/INPUT.md at main · afollestad/material-dialogs
material-dialogs/documentation/INPUT.md at main · afollestad/material-dialogs
😍 A beautiful, fluid, and extensible dialogs API for Kotlin & Android. - afollestad/material-dialogs
github.com
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingLeft="@dimen/md_dialog_frame_margin_horizontal"
android:paddingRight="@dimen/md_dialog_frame_margin_horizontal"
android:paddingBottom="@dimen/md_dialog_frame_margin_vertical_less">
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/md_input_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/md_input_message"
android:layout_width="match_parent"
android:layout_height="wrap_content"
tools:text="Hello, World!" />
</com.google.android.material.textfield.TextInputLayout>
</FrameLayout>
5. 해당 Activity 의 theme 수정
위에서 말한 xml layout 을 활용 하기 위해서는 사용하는 activitify 의 테마 정보도 제약이 있습니다. 아래 내용으로 지정 하는 않으면 build 하는 과정에서 오류 메시지가 나타나게 됩니다. 아래의 예시에서 참고할 부분은 parent theme 로 지정된 Theme.AppCompat 으로 지정하는 않으면 build 과정에서 오류가 발생 됩니다. 그리고 manifest 파일에서 해당 activity 의 theme 도 아래 name 의 값으로 지정해 주어야 합니다.
<?xml version="1.0" encoding="utf-8"?>
<resources>
<style name="Theme.Multichat416" parent="Theme.AppCompat" >
<item name="fontFamily">@font/notosanskr_regular</item>
<item name="md_color_title">@color/white</item>
<item name="md_color_content">@color/white</item>
<item name="md_color_button_text">@color/white</item>
<item name="md_background_color">@color/softBlue</item>
<item name="md_ripple_color">@color/white</item>
<item name="md_divider_color">@color/softBlue</item>
<item name="md_font_button">@color/softRed</item>
<item name="md_item_selector">@color/softBlue</item>
</style>
</resources>
마무리
이렇게 해서 Jetpack Compose와 com.afollestad.material-dialogs 라이브러리를 사용하여 비밀번호 입력 다이얼로그를 구현하는 방법에 대해 알아보았습니다. 이 예제는 기본적인 다이얼로그 구현 방법을 보여주며, 필요에 따라 다이얼로그의 디자인과 동작을 커스터마이즈할 수 있습니다.
이 글이 도움이 되었기를 바랍니다! 질문이나 의견이 있으시면 댓글로 남겨주세요. 감사합니다!
'모바일 앱(안드로이드)' 카테고리의 다른 글
Jetpack Compose를 사용하여 AdMob 배너 광고 추가하기 (0) | 2025.02.01 |
---|---|
Android Studio를 사용하여 Google Maps 연동 앱 만들기 (1) | 2025.01.30 |
Android studio 의 AVD의 화면은 왜 블랙 스크린이 되는 걸까 ? (3) | 2025.01.22 |
Jetpack Compose를 활용한 채팅방 구현 및 MutableStateFlow 사용법 (0) | 2025.01.18 |
Android Kotlin으로 동적인 다국어 번역기 앱 만들기 (1) | 2025.01.16 |